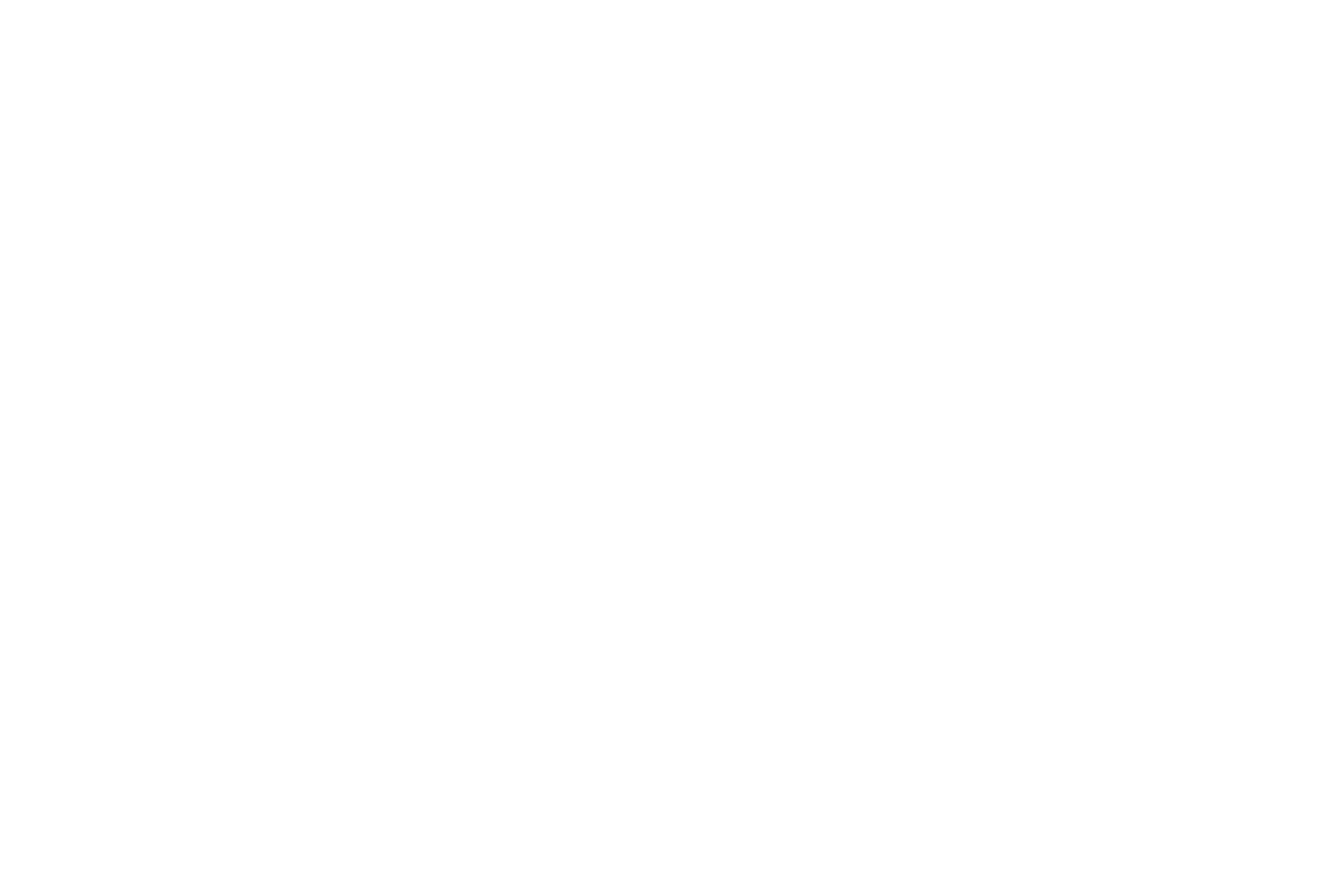
Make AI Understand Your Codebase
Sequa turns every repo, doc and ticket into a live knowledge graph so Cursor, Copilot & Windsurf finally "get" your project.
Make Cursor 2× Smarter
Sequa deeply understands your project, so your AI stack always knows the full story.
Frequently Asked Questions
Talk to a Founder
We'd love to hear from you! Have questions, ideas, or just want to connect? Drop us a message and we'll get back to you personally.